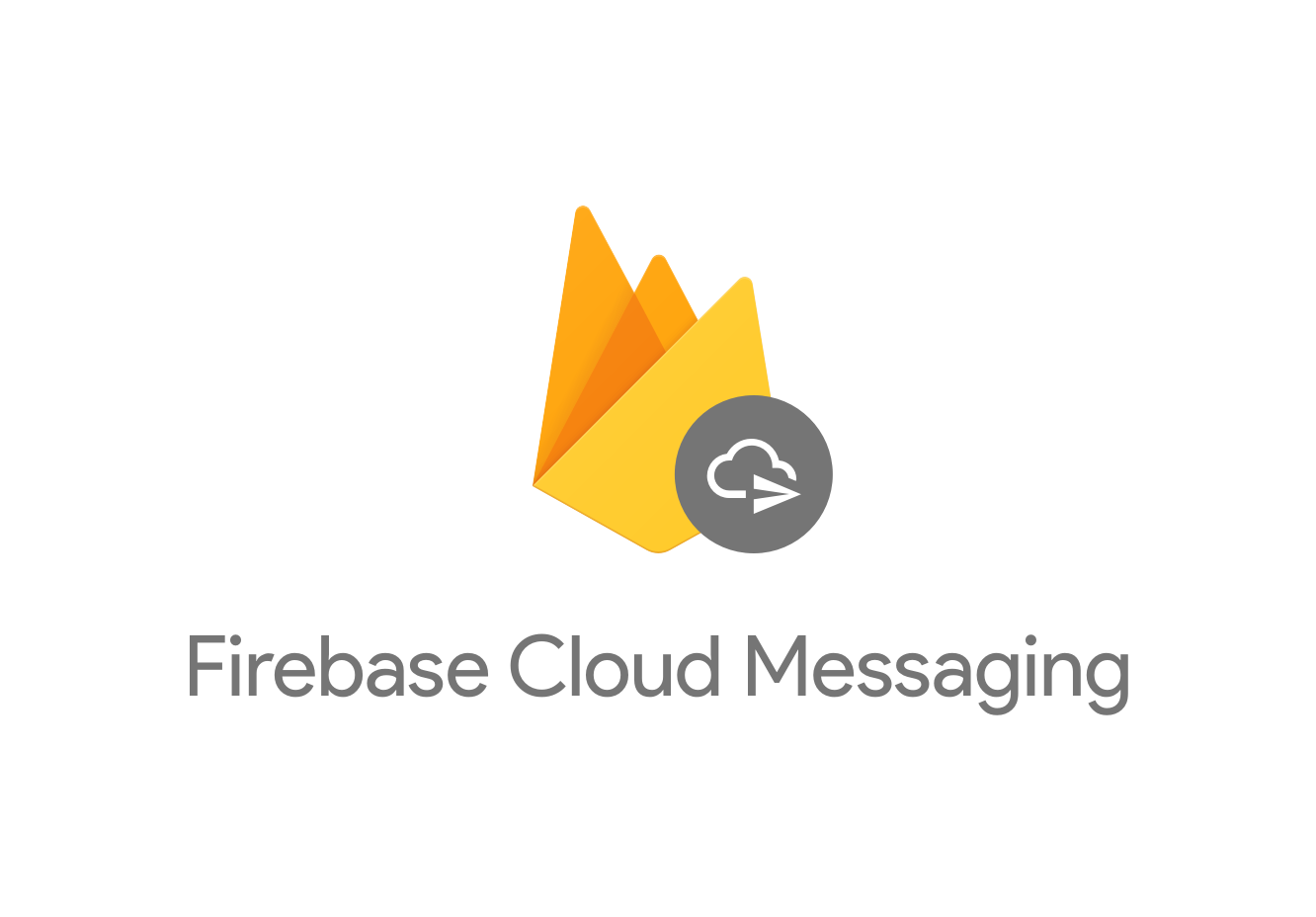
Built with Firebase
Firebase Cloud Messaging
webpushのプロトコルを使って、ブラウザやモバイルアプリなどのクライアントにメッセージを配信してくれるクラウドサービス
WebPushとは
ブラウザにServiceWorkerというプログラムを登録する。
あるサイトを訪れたときに、「このサイトから通知を受信しますか?」みたいなメッセージが出ることがあると思う。ここで許可すると、サイトからServiceWorkerスクリプトをダウンロードしてブラウザに登録される。
ServiceWorkerはブラウザの裏で常に動いていて、Webアプリケーションと連携していろんな処理を行うことができる。その一つがプッシュ通知の受信。
通知を送る側のWebアプリケーションは、メッセージ配信サーバーを通じて通知を送る。
ServiceWorker登録時には、勝手にメッセージ配信サーバーに対してSubscribe(購読)というものを行っていて
メッセージ配信サーバーは、送信元Webアプリの通知をSubscribe(購読)しているServiceWorkerに対してメッセージを送信する。
ServiceWorkerがメッセージを受け取ると、ブラウザ上でポップアップが出てきて通知してくれる。
Youtubeの配信開始通知などがそれ。
で、上で登場したメッセージ配信サーバーにあたるサービスの一つが、FCM(Firebase Cloud Messaging)
※Webpushの仕組みは正直ややこしいので間違っているかも
chrome拡張の中でWebPushを使いたい
ネット上にchrome拡張とFCMとの連携ナレッジがあまりないが、
以下の感じでいける
以下にサンプルとして、いま自分が作っているchrome拡張のコードを一部抜粋してみる。
ProjectRoot
|-manifest.json
|-background.html
|-background.js
|-firebase-messaging-sw.js
Firebaseのプロジェクト登録
ここを参考にFirebaseでプロジェクトを作成し、アプリ登録まで行う。
プロジェクトの設定画面
- 全般 の画面で
Firebase SDK snippetを探し
var firebaseConfig = {...}
の中身をメモっときます。
あとでchrome拡張の中で使います。
プロジェクトの設定画面
- CloudMessaging の画面で
ウェブプッシュ証明書からキーペアを生成する。
「鍵ペア」として表示されてるランダム文字列が、クライアントが使う公開鍵なのでこれもあとで使います。メモっておく。
chrome拡張の作成
細かいchrome拡張の作り方は今回は省く
そのうち別記事にしたい
manifest.json
{
"name": "Stream Sync",
"version": "0.1",
"description": "Sync the timing of streaming simultaneously, between host and clients.",
"permissions": ["storage", "activeTab", "declarativeContent", "notifications"],
"background": {
"page": "background.html",
"persistent": true
},
"page_action": {
"default_popup": "popup.html"
},
"content_security_policy": "script-src 'self' https://www.gstatic.com/ https://*.firebaseio.com https://www.googleapis.com; object-src 'self'",
"manifest_version": 2
}
permissions
にnotifications
を追加しておくこと。これによりユーザーの許可なしにServiceWorkerを登録できる。content_security_policy
に、firebaseライブラリのインポート元ホストを指定しておく。chrome拡張の場合、必要らしい。- その他のオプションはそれぞれのchrome拡張で自由に
backgroundスクリプト
<!-
- background.html -->
<html>
<head>
<meta charset="utf-8"/>
<title>StreamSync Background</title>
</head>
<body>
<script src="https://www.gstatic.com/firebasejs/8.1.1/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/8.1.1/firebase-messaging.js"></script>
<script src="background.js"></script>
</body>
</html>
//background.js
// Initialize Firebase
var firebaseConfig = {
// firebaseコンソールからコンフィグをコピペする
};
firebase.initializeApp(firebaseConfig);
const messaging = firebase.messaging();
const setMessagingToken = async (vapid) => {
const registration = await navigator.serviceWorker.register('firebase-messaging-sw.js');
//オプションのserviceWorkerRegistrationは任意。指定しない場合デフォルトでプロジェクトルートの firebase-messaging-sw.jsをServiceWorkerとして登録する。
const currentToken = await messaging.getToken({
'serviceWorkerRegistration': registration,
'vapidKey': vapid,
});
if(currentToken) {
console.log('got token');
// 受け取ったtokenをchromeAPIのstorageに保存しておこう
chrome.storage.local.set({'messagingToken': currentToken}, () => {
console.log('successful to get messaging token');
});
alert(currentToken); // tokenをモーダルで表示。通知を送信する際に使う。
} else {
console.log('failed to get messaging token');
chrome.storage.local.remove('messagingToken', undefined);
}
}
chrome.runtime.onInstalled.addListener(function() {
setMessagingToken( {{自分のvapidKey}} );
});
- firebaseConfigは先ほどメモったものをコピペする
- vapidKeyは先ほどメモった文字列をコピペする
ServiceWorkerスクリプト
// firebase-messaging-sw.js
importScripts('https://www.gstatic.com/firebasejs/8.1.1/firebase-app.js');
importScripts('https://www.gstatic.com/firebasejs/8.1.1/firebase-messaging.js');
firebase.initializeApp({
// firebaseコンソールからコンフィグをコピペする
});
const messaging = firebase.messaging();
messaging.setBackgroundMessageHandler(payload => {
const notificationTitle = 'Background Message Title';
const notificationOptions = {
body: 'Background Message body.',
icon: '/firebase-logo.png'
};
self.registration.showNotification(notificationTitle,notificationOptions);
});
メッセージ送信テスト
chrome拡張のインストール
- chromeの拡張機能画面へ
- デベロッパーモードon
- パッケージ化されていない拡張機能を読み込む
- 作成したchrome拡張のプロジェクトフォルダを指定
backgroundスクリプトを上の通り作っていると、拡張機能のインストール時にアラートでFCM用のトークンが表示される。
テストメッセージ送信の際の"宛先"となるので、これをメモっておく。
firebaseコンソールからテストメッセージの送信
firebaseコンソールの左タブから、Cloud Messagingへ
新しい通知 を選択
(初めての場合は「初めての通知を送信する」?)
通知の作成画面で
タイトル、テキストを入力し テストメッセージを送信
「FCM登録トークンを追加」に先ほどのトークンを指定
送信
問題なければ、通知のポップアップが出るはず